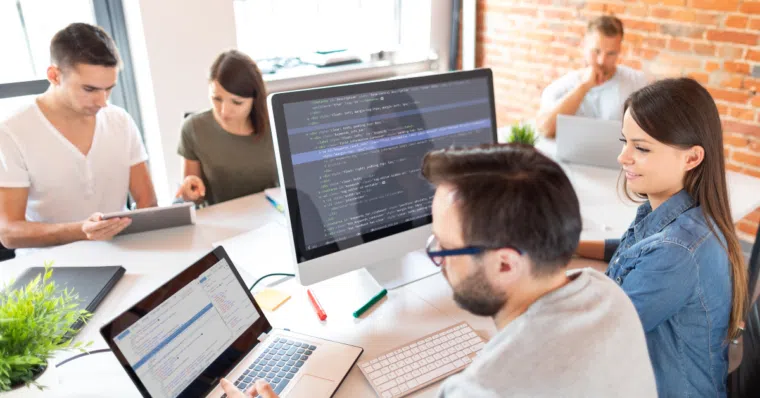
Customizing Webpack Configurations for Full-Stack Projects
If you are building modern web applications, you have likely heard about Webpack. It is a powerful tool used in many full-stack projects to bundle JavaScript, CSS, and other files together. But Webpack is more than just a tool for combining files — it can also be customized to make your full-stack project faster, smarter, and easier to manage.
In this blog, we will explore how to customize Webpack configurations for full-stack projects. If you are learning through a developer course, or you are currently taking a full stack developer course in Hyderabad, this guide will help you understand Webpack and how to make it work for your specific needs.
Let’s start with the basics and then move into simple ways to customize Webpack for both frontend and backend development.
What Is Webpack?
Webpack is a module bundler. It takes your project files — JavaScript, CSS, images, and more — and bundles them into one or more files that the browser can understand.
In a full-stack application, especially when you use tools like React for the frontend and Node.js for the backend, Webpack helps you organize and build your frontend code efficiently.
When you customize Webpack, you can:
- Improve build speed
- Add support for different file types
- Use environment variables
- Split code into smaller parts
- Reduce the final file size
Now, let’s look at how you can customize Webpack step by step.
Basic Webpack Setup
To use Webpack, you need to install it first:
npm install webpack webpack-cli –save-dev
Then, create a file named webpack.config.js in the root of your project.
Here is a very basic example of a Webpack config file:
const path = require(‘path’);
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
mode: ‘development’,
};
This tells Webpack:
- Entry point: where your code starts
- Output: where to put the final bundled file
- Mode: development (for debugging) or production (for deployment)
This basic setup works well, but for full-stack projects, you may need more customization.
Using Loaders in Webpack
Loaders help Webpack understand other file types like CSS, images, and JSX (used in React).
Here’s how you can add loaders:
JavaScript and JSX:
Install Babel loader:
npm install babel-loader @babel/core @babel/preset-env @babel/preset-react –save-dev
Update webpack.config.js:
module: {
rules: [
{
test: /\.(js|jsx)$/,
exclude: /node_modules/,
use: {
loader: ‘babel-loader’,
},
},
],
}
CSS and SCSS:
Install CSS loaders:
npm install style-loader css-loader –save-dev
Update config:
{
test: /\.css$/,
use: [‘style-loader’, ‘css-loader’],
}
If you are learning this in a developer course, your trainer may have already explained how loaders work. It’s a good idea to try them yourself in a small project.
Setting Up Webpack for React
When using React, you often need to support JSX, import CSS files, and load images. You can do all of this with Webpack.
Here’s a basic webpack.config.js for a React frontend:
const path = require(‘path’);
module.exports = {
entry: ‘./src/index.jsx’,
output: {
path: path.resolve(__dirname, ‘dist’),
filename: ‘main.js’,
},
mode: ‘development’,
module: {
rules: [
{
test: /\.(js|jsx)$/,
exclude: /node_modules/,
use: [‘babel-loader’],
},
{
test: /\.css$/,
use: [‘style-loader’, ‘css-loader’],
},
],
},
resolve: {
extensions: [‘.js’, ‘.jsx’],
},
};
This configuration allows you to:
- Write React components using JSX
- Import CSS files directly in your JavaScript
- Bundle everything into one output file
This is very useful when building the frontend part of your full-stack app.
Customizing Webpack for Development and Production
You can use different Webpack configurations for development and production. This helps you have a better development experience and smaller build files when deploying.
Step 1: Create Two Config Files
- webpack.dev.js for development
- webpack.prod.js for production
Step 2: Common Configuration
Create a webpack.common.js for shared settings.
module.exports = {
entry: ‘./src/index.jsx’,
output: {
filename: ‘main.js’,
path: path.resolve(__dirname, ‘dist’),
},
resolve: {
extensions: [‘.js’, ‘.jsx’],
},
};
Step 3: Use Webpack Merge
Install webpack-merge:
npm install webpack-merge –save-dev
Now you can merge the common config with dev and prod configs.
Example: webpack.dev.js
const { merge } = require(‘webpack-merge’);
const common = require(‘./webpack.common.js’);
module.exports = merge(common, {
mode: ‘development’,
devtool: ‘inline-source-map’,
});
Example: webpack.prod.js
const { merge } = require(‘webpack-merge’);
const common = require(‘./webpack.common.js’);
module.exports = merge(common, {
mode: ‘production’,
});
This structure is useful if you want to add special plugins or optimizations for production.
If you’re enrolled in a developer course in Hyderabad, ask your mentors how to implement multi-environment builds using Webpack.
Adding Plugins for Better Builds
Webpack has many plugins that make your build better. Here are a few common ones:
HtmlWebpackPlugin
Automatically creates an HTML file with your bundled JavaScript.
Install it:
npm install html-webpack-plugin –save-dev
Use it in webpack.config.js:
const HtmlWebpackPlugin = require(‘html-webpack-plugin’);
plugins: [
new HtmlWebpackPlugin({
template: ‘./public/index.html’,
}),
],
CleanWebpackPlugin
Removes old files from the output folder before each build.
Install:
npm install clean-webpack-plugin –save-dev
Use in config:
const { CleanWebpackPlugin } = require(‘clean-webpack-plugin’);
plugins: [
new CleanWebpackPlugin(),
],
These plugins make your build clean and automatic.
Using Webpack with a Backend
In full-stack apps, you usually have both frontend and backend code. Webpack is mostly used for the frontend, but you can also use it with backend Node.js apps if needed.
For example, you can set up Webpack to:
- Transpile server-side code using Babel
- Bundle backend utilities or shared code
- Load environment variables using dotenv
However, in most full-stack projects, you keep the backend separate and use Webpack only for frontend code.
In a full stack developer course, you will learn how to connect frontend and backend using APIs, and how Webpack helps in deploying the frontend part smoothly.
Webpack Dev Server for Fast Development
Webpack also has a dev server that lets you see changes in real time as you code.
Install it:
npm install webpack-dev-server –save-dev
Add it to your config:
devServer: {
static: ‘./dist’,
port: 3000,
open: true,
hot: true,
},
Now run your app using:
npx webpack serve
It will open in the browser and reload when you save your code.
Final Thoughts
Webpack is a powerful tool for full-stack development. It helps you bundle, optimize, and manage frontend assets. With a few simple customizations, you can make your full-stack project faster and cleaner.
If you are learning from a developer course in Hyderabad, use this opportunity to understand Webpack well. Practice customizing configurations and adding loaders and plugins. These skills will help you in real-world projects and job interviews.
To summarize:
- Start with a basic Webpack setup
- Add loaders for JavaScript, CSS, and images
- Split configs for development and production
- Use plugins for automation
- Learn how to use Webpack with React and backend APIs
- Use Webpack Dev Server for faster development
With practice and patience, you’ll master Webpack and become a better full-stack developer.
Contact Us:
Name: ExcelR – Full Stack Developer Course in Hyderabad
Address: Unispace Building, 4th-floor Plot No.47 48,49, 2, Street Number 1, Patrika Nagar, Madhapur, Hyderabad, Telangana 500081
Phone: 087924 83183